SE-0010 Introduction to Java
Lab 2: Interest Calculator
Outcomes
- Develop additional proficiency with the Java language
- Develop further proficiency using common Java classes, such as
Math, SImpleDateFormat, DecimalFormat, etc.
- Program mathematical expressions and
formulas using the Java Math package
- Use methods of the String class to manipulate String data
- [Optional] Incorporate conditional control
statements, such as if and switch, into a Java program to affect
program control flow.
Assignment
For this assignment, you will write a program that
computes the value of an interest-bearing CD (Certificate of Deposit) over a
period of time. The initial value of the CD, the interest rate, and the period
of time will all be determined by asking the user for those values.
Your program will consist of a single
InterestCalculator class. For this assignment, this will be the only class
needed. Within this class, you'll use several methods, as follows:
main method - this is the usual method where your
program begins to execute when it is run, from within this method, you'll print
a JOptionPane-based welcome screen, and after that, you'll call the following
methods:
getInitialValue - this is a method that prompts the
user for the initial value of the CD, converts that input to a numerical value,
and returns that value to main().
getInterestRate - this is a method that prompts the
user for the annual interest rate of the CD, converts that input to a numerical
value, and returns that value to main().
getInterval - this is a method that prompts the
user for the intervals (in years) at which the compounded value of the CD will
be computed; it converts that input to a numerical value, and returns that value
to main()
computeCDValue - this is the method that computes
the value of the CD at a certain period of time (in years) after the original
purchase. This method expects three arguments: the original purchase price of
the CD, the annual interest rate, and the number of years since the CD was
purchased. It computes the compounded value and returns that value to main().
From main(), you'll call this method 5 times, computing the value at 5 different
intervals after purchase and storing the results in five different variables.
For example, if the user entered 3 as the interval value, then you'll compute
and store the CD compounded values at 3, 6, 9, 12, and 15 years after the
original purchase date.
displayResults - this is the method that displays
the results of the computations. This method expects five arguments: the five
computed results of the compounded values of the CD at the five intervals. This
method returns nothing back to main().
After you display the results, your program should end.
Detailed requirements
- Your program is to present to the user a JOptionPane-based
welcome message stating what it does and what it will ask the user for in terms
of information, similar to that shown below:
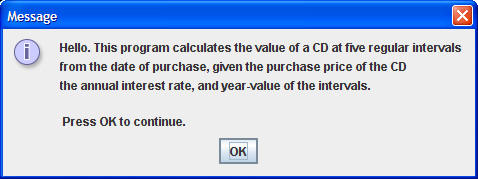
- Your program must prompt the user for three pieces of
information:
- The purchase price of the CD. You must
accept a value such as 1000.00, representing $1000.00.
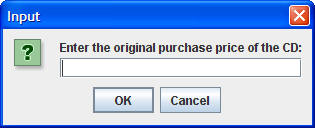
- The interest rate which the CD bears. You must
accept a value such as 7.6, representing 7.6%.
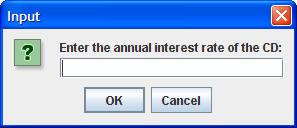
- The year-intervals at which the compounded value
of the CD will be computed. You must accept a value such as 3,
representing 3 years.
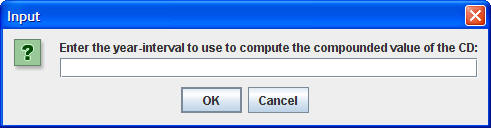
- If interest on the CD is compounded daily, the formula
for calculating the compunded value of the CD is given by
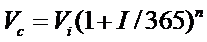
where
Vc is the current value,
Vi is the initial value,
I is the annual percentage rate (note that I is 0.076 if the
interest rate is 7.6%),
n is the number of days (not years) since the purchase of the
CD. Assume every year has 365 days (which is not exactly true, but it's
close enough).
- You must use JOptionPane.showMessageDialog to output
the information in a neatly
formatted fashion. The output should appear as follows (example output is for
a $1000 CD bearing 5% interest, computed for five 2-year intervals):
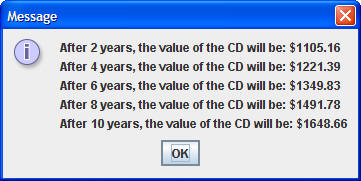
- You must output the monetary value with 2
decimal places of precision, as shown above.
- [Optional] When the user enters the purchase price of the
CD,
you should ensure that the value is greater than 0, and if it is not, inform the
user of the error via a JOptionPane-based dialog that informs the user
that the purchase price must be specified as a value greater than 0.0.
- [Optional] When the user enters the interest rate for the
CD,
you must ensure that the value is greater than 0 and less than 100, and if it
is not, inform the user of the error via a JOptionPane-based dialog that
informs the user that the interest rate must be specified as a value between
0.0 and 100.0.
- [Optional] When the user enters the
year-interval for computing the compounded values,
you must ensure that the value is greater or equal to 1 and less than or equal
to 10, and if it
is not, inform the user of the error via a JOptionPane-based dialog that
informs the user that the year-interval must be specified as a value between
1 and 10.
- [Optional] In all error cases, after you display the message
dialog, you should terminate the program by calling System.exit(0).
Test your program and make certain that it works
correctly; use the results shown above to verify your output.
Remember: This is an individual assignment. While you may
discuss the concepts with your classmates, you must write your own program. You
may not copy or borrow code from your classmates.
Lab Submission (due 8:00am, Tuesday following
lab)
Submit your completed Java program via via
WebCT
(assignment "Lab 2: Interest Calculator").. For this
program, your program will consist of a single .java file.
Be sure to keep a copy of your work, in case something gets lost.
Your grade will be based on the following criteria:
- Meeting requirements - that is, a working Java
program..
- Technical quality of both your program. For the program, technical quality consists of formatting,
commenting, and following coding style guidelines we discussed in lectures
and the textbook.
- Spelling and grammar (in comments).
- Timeliness of submission as stated in the
course policies.