SE-1011 Software Development 1
Lab 5: Interest Calculator
Objectives
- continue to develop proficiency with the Java language
- Program mathematical expressions and
formulas, with the aid of the Java Math class
- Use methods of the String and JOptionPane classes to
output data in a specific format
- Incorporate conditional logic statements, such as if and
else if, into a Java program to affect control flow.
- Incorporate iteration statements, such as do-while
and while, to repeat specific instructions.
Assignment
Scenario: You have discovered that, unknown to you, a distant relative
purchased a Treasury bond for you on the day you were born. Unfortunately, you
don't yet know how much it is worth. Will you be able to buy that
Ferrari? or will you have to settle for a 1983 mini-van? In order to find out, you need to write a
Java program that computes the
value of the bond at various intervals, from its initial value to its value
after some specified number of years.
Detailed requirements
In this lab, you will write a program that computes the
changing value of a Treasury bond based on its initial value and % rate of return. You
will incorporate conditional
control statements (if-else if-else) into your program in order to provide a basic error handling mechanism for invalid inputs entered
by the user. You'll also incorporate iteration statements (while, or do-while)
to automatically compute the bond value at various intervals.
After reading through the following requirements, draw a
flowchart (it will be more significantly more complex than any you've drawn
previously). This will greatly help you in figuring out the steps needed in the
actual implementation.
Your program is to present to the user a JOptionPane-based
welcome message stating what it does and what it will ask the user for in terms
of information, similar to that shown below (but you can customize your welcome
message):
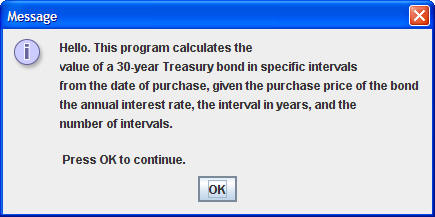
- Your program's must then prompt the user for four pieces of
information:
- The purchase price of the Treasury bond. You must
accept a value such as 1000.00, representing $1000.00.
- The interest rate which the bond bears. You must
accept a value such as 7.6, representing 7.6%.
- The interval (in years), at which the bond value is
calculated.
- The number of intervals (a positive integer) for
which to perform the calculations.
Interest on the bond is compounded daily, and formula
for calculating the current value of the bond is given by
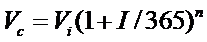
where
Vc is the current value,
Vi is the initial value,
I is the annual percentage rate (note that I is 0.076 if the
interest rate is 7.6%),
n is the number of days since the purchase of the
bond.
- The Math.pow() method can be used to
compute the value of a number raised to a power; for example:
double x = 2;
double y = 3;
double z = Math.pow(x,y); // computes 2 to the 3rd and places the result (8)
in z.
- You must use JOptionPane.showInputDialog
and JOptionPane.showMessageDialog to input and output information in a neatly
formatted fashion. The output should appear as follows for a purchase price of
$1000.00, an interest rate of 5%, an interval of 3 years, and 4 intervals:
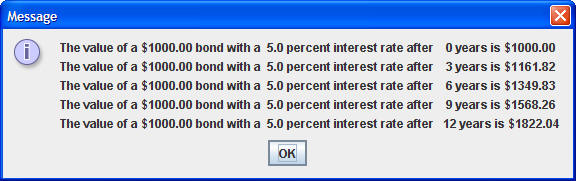
- You must output the monetary values with 2
decimal places of precision, as shown above. See hints below on how to format
this information.
- You must output the interest rate with 1
decimal place of precision, as above.
- When the user enters the purchase price of the bond,
you must ensure that the value not null, not empty, and is greater than 0. If it is not, inform the
user of the error via a JOptionPane-based dialog that informs the user
that the purchase price must be specified as a value greater than 0.0.
- When the user enters the interest rate for the bond,
you must ensure that the value is not empty, is greater than 0 and less than 100.
If it
is not, inform the user of the error via a JOptionPane-based dialog that
informs the user that the interest rate must be specified as a value between
0.0 and 100.0.
- When the user enters the interval,
you must ensure that the value is not empty, and is greater than 0. If it
is not, inform the user of the error via a JOptionPane-based dialog that
informs the user that the interval must be greater than 0.
- When the user enters the number of intervals,
you must ensure that the value is not empty, and is greater than 0. If it
is not, inform the user of the error via a JOptionPane-based dialog that
informs the user that the number of intervals must be greater than 0.
- The case of pressing Cancel must not be
treated as an error. Instead, terminate the program if the user presses
Cancel for any of the dialogs.
- In all error cases, after you display an error
message dialog, use iteration to loop back to the prompt for the
specific input.
- When all input has been determined to be valid, use
iteration to loop through the needed calculations repeatedly, until the
number of intervals has been exceeded.
- Every conditional test must be fully
commented to let the reader know what you are doing in the case where the
if condition is satisfied, as well as the case where the else or
else if condition is satisfied instead.
- Name your class InterestCalculator;
declare the package as msoe.se1011.<your msoe email name>
Hints:
- You can use the String class's
format() method to create a formatted String. For example to create a
String named output and set its content to be "The value of x is
2 and the value of y is 3.50", you'd write:
String output;
int x = 2;
double y = 3.5;
output.format("The value of x is %4d and the value of y is %6.2f \n", x, y
);
depending on the values of x and
y, of course, the contents of the String output would be
different than that above. Note that the format specification used by the
format() method is similar to that used in the printf() method
discussed in class.
- Remember that you can concatenate Strings
together using the + operator. As an example:
String output;
String message = ""; // initially empty
int x = 2;
double y = 3.5
output = String.format("The value of x is %4d and the value of y is %6.2f \n", x, y
);
message += output; // concatenate the strings
x = 4;
y = 1.2;
output.format("The value of x is %4d and the value of y is %6.2f \n", x, y
);
message += output; // concatenate again
At the end, the contents of the String
message would be:
"The value of x is 2
and the value of y is 3.50
The value of x is 4 and the value of y is 1.20
"
Lab Submission (due by end of lab)
Submit your assignment following these instructions:
- Hand in your flowchart to your instructor.
- Upload your .java file through
WebCT
(assignment "Lab 5: Interest Calculator").
Be sure to keep copies of all your java files, in case something gets lost.
Your grade will be based on the following criteria:
- Meeting requirements; your program must compile and
run. If you have trouble, you must come to see me. If you don't and you
submit a program that does not compile or produce meaningful results will
receive a grade of no higher than 50, even after reworking your program.
- Technical quality of your program. Technical quality consists of formatting, commenting, and
following coding style guidelines we discussed in lectures.
- Spelling and grammar (Eclipse even has a built-in spell
checker - make use of it!)
- Timeliness of submission as stated in the
course policies.