SE-1021 Software Development 2
Lab 4: Barnyard fun with inheritance
Outcomes
- Use inheritance and polymorphism to create classes that
exhibit similar interfaces and behaviors
Assignment
You will write a program that makes use of a number of supplied classes. In
addition, you will define an interface and modify some of the supplied classes
to implement the interface. Your program will generate a random number of
barnyard animal objects. The animal objects will then make sounds and calculate
their market value.
Project Setup
- First create the Lab4 project. Download this
lab4.zip
file; then follow these steps to import it :
- With the Lab4 project selected in the Package Explorer
select File -> Import...
- From the Import dialog select General -> Archive File
and click Next
- Click the first Browse button to and select the
lab4.zip file you downloaded
- When you have selected the lab4.zip
file click Open and then Finish to complete the import.
You will see a folder named src in your
project; right-click this folder, and select Build Path/Use this
source folder from the context menu. Once part of the build path,
you'll see two packages: edu.msoe.se1021.audio
and edu.msoe.se1021.YOURLOGIN.
- Rename the edu.msoe.se1021.YOURLOGIN
to match your MSOE username (see steps below). Do not modify the
edu.msoe.se1021.audio package name.
- Select the package name in the package explorer
- Right-click and select Refactor -> Rename from the context
menu
- Enter the new name and click OK
The lab4.zip file includes the following
interface and classes:
- Vocalize.java -- An interface for
objects that can make sounds (vocalize).
- Livestock.java - An abstract class that implements the behavior
and attributes common to all barnyard animals.
- Dog.java -- Represents a dog and
implements the Vocalize interface.
- Sheep.java -- Represents a sheep
and implements the Vocalize interface.
- Horse.java -- Represents a horse
and implements the Vocalize interface.
- Chicken.java -- Represents a
chicken and implements the Vocalize interface.
- WavPlayer.java -- Loads and plays a
.wav file.
The WavPlayer.java
class and Vocalize.java interface
(shown in gray in the UML class diagram below) are already complete and do not
have to be modified for this assignment.
The 'animal' java files (shown in tan) are mostly complete; you'll have to
modify them as described below in Parts 2 and 3.
You'll have to create two files from scratch:
Lab4.java and MarketPrice.java
(also in tan). These are described in Parts 1-3 below.
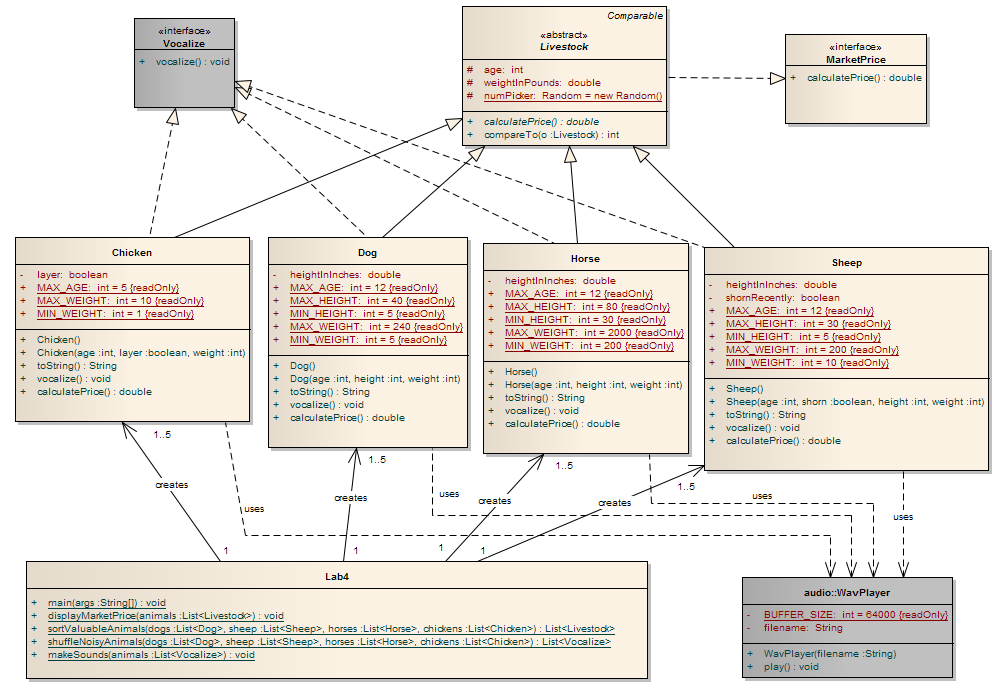
Part 1: Make sounds (to be demonstrated before the end
of lab)
You must demonstrate proper functionality for Part 1 before the end of lab
(worth 15 points).
Create a Lab4 class (in part 1, do not modify
any other class or interface files) that contains the following class (i.e.,
static) methods (consult the UML class diagram and follow it when
declaring the methods below) :
main() -- Contains the program.
Specifically, it should:
- Create an ArrayList<> for each type
of barnyard animal (dog, sheep, horse, and chicken). Each
ArrayList<> should contain a random
number (between one and five) of objects (see
Random class).
- Call shuffleNoisyAnimals to get one
List<> containing references to objects
that implement the Vocalize interface.
- Pass the returned List<> to
makeSounds() to cause each animal to
vocalize (play its sound).
shuffleNoisyAnimals -- Accepts four
Lists<> (one of each type of barnyard animal)
and creates one ArrayList<> containing all of the barnyard animals.
- The ArrayList<> must be declared
such that it may contain references only to objects that implement
the Vocalize interface.
- The Collections.shuffle() method
must be used to randomize the order of the barnyard animals.
makeSounds -- Accepts a
List<> of
Vocalize objects and causes each object to vocalize.
Javadoc for the Lab4 class upon completion of
Part 3 is available here and may prove to be a
useful reference for Part 1 as well.
Demonstrate this working program to your instructor before the end of the
lab.
Part 2: Implement market price calculation
In addition, you must define a MarketPrice
interface for objects that can determine their market price. Be sure to follow
the specification illustrated in the UML class diagram, where the abstract
Livestock class inherits this interface, which is then "passed through" to
the subclasses. You will then need to modify the barnyard animal classes so that
they actually implement the MarketPrice
interface method. The market price for each type of barnyard animal is
determined as follows:
Market Price for Dog
$500 / (age+1)
Market Price for Sheep
The base price is: $1.05 * (weight in pounds).
If the sheep hasn't been shorn recently, the following premium should be
added to the price: $0.40 * (height in inches).
Market Price for Horse
If the horse is less than 80% of the maximum age for a horse, then the market
price is determined by: $1000 * (the percentage of life remaining for the
horse). You should assume that the horse lives to the maximum age of a horse
(as specified within the Horse class).
Otherwise, the market price is: $0.12 * (weight in pounds).
Market Price for Chicken
If the chicken is a layer, the market price is: $1 * ( (maximum chicken
age) - (age) ).
Otherwise, the market price is: $0.38 * (weight in pounds).
Part 3: Sorting by Market Price - implementing the
Comparable<> interface
Once Part 2 is complete, add the following additional functionality to your
program (consult the UML class diagram and follow it when declaring the methods
below):
- main -- Add the following to the
end of the main method written in Part I:
- Call sortValuableAnimals to
get one List<> containing
references to objects (sorted by price, from lowest to highest) that implement the
MarketPrice interface.
- Pass the returned List<> to
displayMarketPrice to cause each
animal to display its market price and details about its attributes.
- sortValuableAnimals -- Accepts four
Lists (one of each type of barnyard
animal) and creates one ArrayList<>
containing all of the barnyard animals. Once created, this method should
call Collections.sort() to re-order the ArrayList<> of animals
sorted by market price. To do this, you'll have to
modify the Livestock class to implement the Comparable<Livestock>
interface so that it implements the compareTo() method of the
Comparable<> interface. This method then returns a List<>
reference to the sorted array ArrayList<>
.
- displayMarketPrice -- Accepts a
List<> of
MarketPrice objects and displays the market price and attributes
of each object.
- You should use the toString
method that is already implemented to display the attributes of each
object.
- The output should look something like this, with prices ascending:
$10.50 for A recently shorn, 2 year old, 28.0 inch tall sheep weighing 10.0 pounds
$62.50 for A 7 year old, 21.0 inch tall dog weighing 18.0 pounds
$500.00 for A 6 year old, 52.0 inch tall horse weighing 1315.0 pounds
- You must format the dollar amounts to always display two
places to the right of the decimal. Hint: you may find the
DecimalFormat and/or
NumberFormat classes useful.
Lab Submission (due date in Blackboard)
- Demonstrate Part 1 to your instructor.
- Create a .zip file of the package
directory containing all of the classes/interfaces in the
edu.msoe.se1021.YOURLOGIN directory (make
sure your Lab4.java file is in this directory
as well).
- Upload your files through
Blackboard
(assignment "Lab 4: Barnyard fun..."). Be sure to keep copies of all your files, in case something gets lost.
Your grade will be based on the following criteria:
- Meeting requirements; your program must compile and
run. If you have trouble, you must come to see me. If you don't and you
submit a program that does not compile or produce meaningful results will
receive a grade of no higher than 50, even after reworking your program.
- Technical quality of your program. Technical quality consists of formatting, commenting, and
following coding style guidelines.
- Spelling and grammar.
- Timeliness of submission as stated in the
course policies.