SE-1021 Software Development 2
Lab 5: Reaction Timer
Outcomes
- implement event-handling for a Graphical User Interface
- implement the ActionEvent interface in a nested inner class
- use the Timer class from javax.swing for generating periodic
ActionEvents
- use the System clock to measure the time between events
Assignment
You will complete the event handler for a program that randomly displays the
names of colors on three different pushbuttons, and instructs the user of the
app to push a button containing a specific color of text. The twist is that the
buttons are randomly labeled "red" , "green", and "blue", but the color of the
button text may be blue, red, and green. This conflict between colors and color
names challenges your brain's ability to separate the text-processing from the
color-processing in order for you to press the correct button. The program also
"times out" after a specified period, after which a new mix of button text and
colors appears. Points are scored for correct button presses, and deducted for
incorrect presses or timeouts.
After 10 attempts, the program displays your total reaction time; that is, the time it
took your brain to process and react to each directive concerning which button
to press.
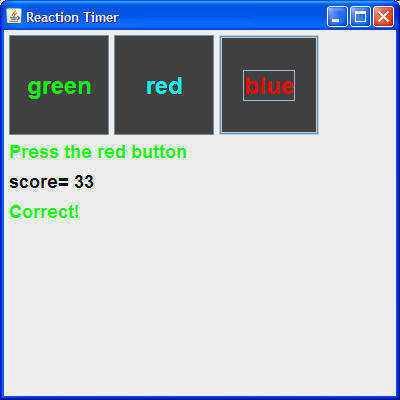
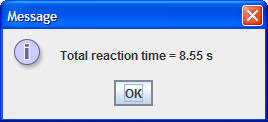
Project Setup
- First create the Lab5 project. Download this
lab5.zip
file; then follow these steps to import it :
- With the Lab5 project selected in the Package Explorer
select File -> Import...
- From the Import dialog select General -> Archive File
and click Next
- Click the first Browse button to and select the
lab5.zip file you downloaded
- When you have selected the lab5.zip
file click Open and then Finish to complete the import.
You will see a folder named src in your
project; right-click this folder, and select Build Path/Use this
source folder from the context menu. Once part of the build path,
you'll see two packages: edu.msoe.se1021.audio
and edu.msoe.se1021.reactiontimer.YOURLOGIN.
- Rename the edu.msoe.se1021.reactiontimer.YOURLOGIN
to match your MSOE username (see steps below). Do not modify the
edu.msoe.se1021.audio package name.
- Select the package name in the package explorer
- Right-click and select Refactor -> Rename from the context
menu
- Enter the new name and click OK
The lab5.zip file includes the following
classes:
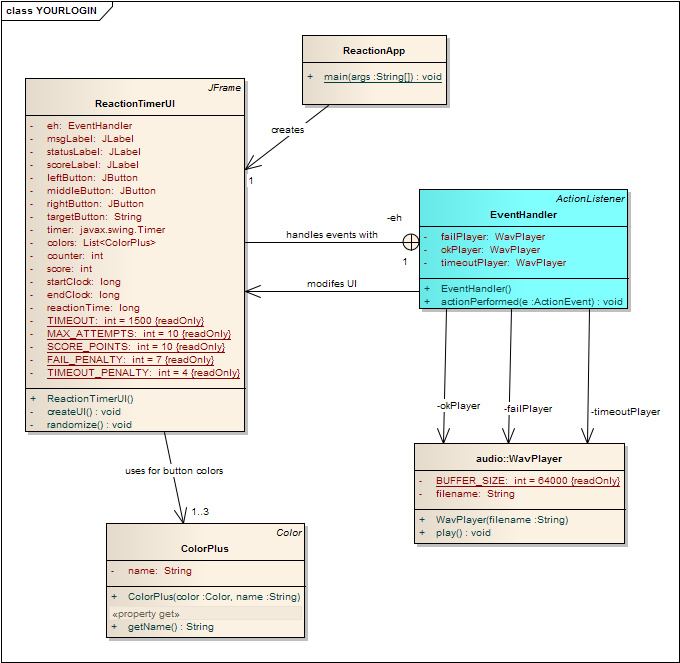
- ReactionApp.java
-- A main class that simply serves as the launch point; it creates the UI.
You do not have to modify this class.
- ReactionTimerUI.java - An JFrame-derived UI class that implements
the user interface. This class itself is complete, but you have to add a nested
inner EventHandler class (shown above in color) to handle events coming from the pushbuttons
of the UI, as well as from the Timer. You also have to read through the
code and comments so that you can understand the various attributes that
you'll have to access/modify from within the EventHandler.
- ColorPlus.java
-- A class that derives from the java.awt.Color class. You do not
have to modify this class.
- WavPlayer.java -- Loads and plays a
.wav file. You do not have to modify this
class.
Details
Follow the TODO comments in the ReactionTimerUI
class. These comments indicate what you have to do to implement the
EventHandler inner class and the event
handling logic.
Lab Submission (due date in Blackboard)
- Demonstrate your Reaction Timer app to your instructor. If you can't do
this by the end of class, you must demonstrate it sometime during Office
Hours before the following lab. You will not receive a grade until you
provide this demonstration. Demonstrations after the due date will be
considered a late submission.
- Upload only your ReactionTimerUI.java file
(containing both the ReactionTimerUI outer and EventHandler
inner classes)through
Blackboard
(assignment "Lab 5: Reaction timer"). Be sure to keep copies of all your files, in case something gets lost.
Your grade will be based on the following criteria:
- Meeting requirements; your program must compile and
run. If you have trouble, you must come to see me. If you don't and you
submit a program that does not compile or produce meaningful results will
receive a grade of no higher than 50, even after reworking your program.
- Technical quality of your program. Technical quality consists of formatting, commenting, and
following coding style guidelines.
- Spelling and grammar.
- Timeliness of submission as stated in the
course policies.