In this class, you will write a program for managing the parts needed to assemble a machine. Your program will provide two ways to view the machine: (1) An assembly guide and (2) a bill of materials.
The assembly guide will give step-by-step instructions for assembling the machine. The bill of materials will give a complete list of the parts and cost of materials needed to build the machine.
To facilitate a wide variety of parts, your program should make use of a generic "Part" interface. This interface will allow generic information about a part to be accessed:
- The part's name
- The total cost of the part
- The total weight of the part
- The bill of materials for a part
There are a few kinds of parts that the system supports: (1) Nuts (2) Bolts (3) SheetMetal (4) Duplicate parts and (5) Assemblies of parts. Nuts, bolts, and sheet-metal are basic parts. Duplicate parts and assemblies are built out of other pre-existing parts.
A class diagram for the program is shown in the figure below:
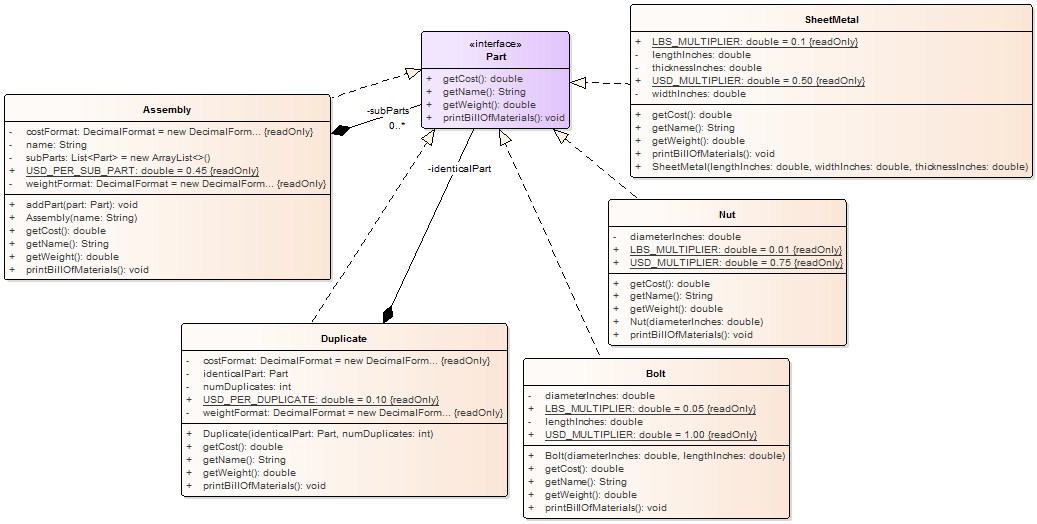
Implement all of the classes shown in the diagram above based on the discussion that follows. The arrows with black diamonds simply indicate that one class has a reference to another, as is already shown in the member variables of the class diagrams for Assembly and Duplicate Part.
A nut costs $0.75 * size^2, where size is the inner diameter of the nut in inches. A nut weights 0.01 lbs * size ^2.
A bolt costs $1 * diameter * length, where diameter is the diameter of the threads in inches and length is the overall length of the bolt in inches. A bolt weighs 0.05 lbs * diameter^2 * length.
Sheet metal costs $0.50 * thickenss * width * height, where each of the dimensions is measured in inches. Sheet metal weighs 0.1 lbs * length * width * thickness.
Bolts, nuts, and sheet-metal do not do anything when printBillOfMaterials is called.
A duplicate part represents count
identical parts. Its cost and weight are both count
times the cost and weight of the single part. However, a duplicate part has an additional $0.10 cost per duplicate part for the labor overhead for managing those extra parts. The duplicate part stores a single reference to another object describing just one of the identical parts.
An assembly consists of multiple different parts. Both the cost and weight of the assembly are determined by adding the cost and weight of each part in the assembly. However, the assembly has an additional construction cost which is $0.45 per sub-part in the assembly. The Assembly maintains a List of all the parts that are stored in it.
A duplicate part or an assembly may be a sub-part of another part. For example, in the example program MachineDriver.java, the cube consists of two parts: a set of metal sheets and several sets of nut-bolt pairs. The sheet-metal is actually a duplicate part, consisting of six identical pieces of sheet metal. The sets of nut-bolt pairs are also a duplicate part, consisting of 36 identical nut-bolt pairs. Each nut-bolt pair is an assembly, consisting of both a nut and a bolt. This hierarchy of parts is illustrated in the diagram below.
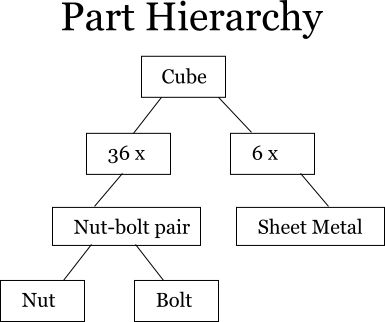
When a duplicate part or assembly prints the bill of materials, it first prints a summary of each part (including name, cost, and weight for each part), then prints the full bill of materials for each part.
For example, the bill of materials for the parts in MachineDriver.java should be printed something like this:
========================== Cube ========================== Part: 36 Nut-Bolt Pairs Cost: $55.69 Weight: 0.247 lbs Part: 6 12.0x12.0x0.25 sheets Cost: $108.60 Weight: 21.6 lbs Total cost: $165.19 Total weight: 21.848 lbs ========================== 36 Nut-Bolt Pairs ========================== Duplicate part: Nut-Bolt Pair Copies: 36 Individual cost: $1.45 Individual weight: 0.007 lbs Total cost: $55.69 Total weight: 0.247 lbs ========================== Nut-Bolt Pair ========================== Part: 0.25x2.0 bolt Cost: $0.50 Weight: 0.006 lbs Part: 0.25inch nut Cost: $0.05 Weight: 0.001 lbs Total cost: $1.45 Total weight: 0.007 lbs ========================== 6 12.0x12.0x0.25 sheets ========================== Duplicate part: 12.0x12.0x0.25 sheet Copies: 6 Individual cost: $18.00 Individual weight: 3.6 lbs Total cost: $108.60 Total weight: 21.6 lbs
As shown in the example above, format numeric values cleanly. In particular, dollar amounts should always include two places after the decimal.