You do not need to write any of the other clases for the milestone.
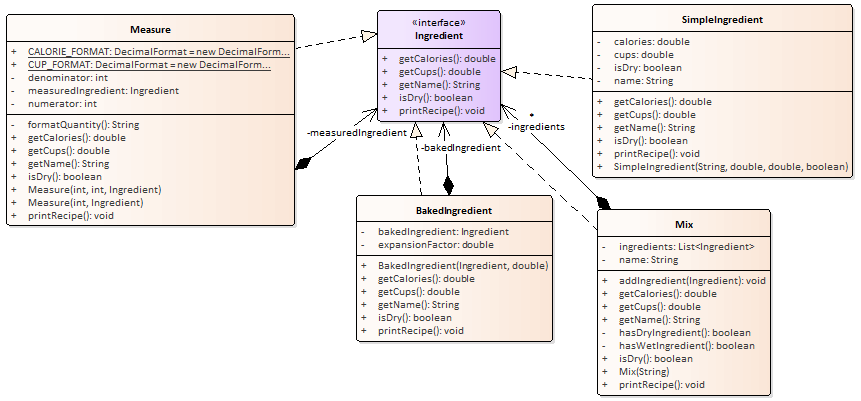
When any of the Ingredient classes print its recipe, it first prints a header containing its name between two lines of equal signs.
## Objectives * Translate UML class diagrams into source code * Construct software using an interface * Employ aggregation and composition in the development of software ## Assignment In this lab, we will explore using interfaces to make deserts. By using interfaces, we can treat prepared ingredients the same as the basic (simple) ones. There are three preparation techniques provided in this lab: (1) baking (2) measuring and (3) mixing. Every ingredient, simple or prepared, provides basic information about itself: * The ingredient's name * The energy content in Calories (kcal) * The volume of the ingredient * A recipe for preparing the ingredient Preparation techniques use existing ingredients to create new ones. ## Assignment Details A class diagram for the program is shown in the figure below:You will upload:
- Your individual .java files
—and— - A README.md file with an informal report (see below)
If you are submiting a draft submission to esubmit (especially near or after the deadline), please include the empty file DONOTGRADE.txt (in big bold capital letters, just like that!) so that I don't start grading something until you intend it to be complete. You can continue making submissions which will be counted as on-time until the deadline or a few minutes after.
Please use the template below for your README.md file. If you create the file from within IntelliJ by right-clicking on your source folder and selecting "new->file", you can just type in README.md, and it will even interpret the markdown for you!
## Introduction [Replace this line with a description of the lab in your own words] ## Conclusion [Replace this line with a description of what you learned in this lab] ## Things I Liked / Suggestions for Improvement [Replace this line with what you liked or think could be improved about the lab. (Required.)]Esubmit will run your code and check your output against the examples given in the lab. Although your output does not need to exactly match the output on esubmit, please make it close and consider whether differences are relavent. For example, a different number of equal signs on the lines does not matter, but making things singular that should be plural and vice-versa could. Exercise your own discression in evaluating these differences. If you see any of the following errors, please resubmit your lab. (You may submit multiple times before the deadline.) If you see this: