This is an old version of this course, from Spring 2015. A newer version is available here.
This assignment is a team assignment. Please work in teams of two unless approved by the instructor.
Equipment Needed
- Your beaglebone black
- 5 V power supply (probably good to get in the habit of using this)
- SE3910 Kit (Checkout from Tech Support)
- USB Oscilloscope (Digilent Analog Discovery)
- Beaglebone Breadboard Cape
- (optional) Beaglebone Breakout Board
- Logitech webcam
- A few 2 or 2.2 KΩ resistors (Checkout from me if not in kit)
- (optional) NMap especially zenmap
- Two network cables, or more if you want to share
Some VM and Linux Tips
VM
Use right-control to excape from the virtual machine.
To open terminal: Applications->Accessories->Terminal
From the browser, to copy the path, press Ctrl-L. The graphical folder crumbline (can't find online definition for this word -- do you have one?) will turn into a simple text editor.
ipconfig/ifconfig
Linux equivalent of ipconfig: /sbin/ifconfig
, or, if the path is set up right, ifconfig
. (I hear that ifconfig
, if it works, may not give as complete results as /sbin/ifconfig
. I haven't confirmed this yet.)
On windows with cygwin, ipconfig | grep "IPv4"
gives a summary of IP addresses.
On linux, use /sbin/ifconfig | grep 'inet addr'
to get the same effect.
With a single cable, an alternative configuration (that doesn't require the "share connection" setting) is to manually set all your devices IP addresses. The bone's IP address can be configured using something like ifconfig eth0 192.168.1.100 netmask 255.255.255.0
, and your computer's address using the Windows GUI (e.g. to 192.168.1.101). You will also need to set your virtual machine's IP address — this is possible to do with the GUI (e.g. to 192.168.1.102).
For a single cable, another alternative is to set up your wireless network to share with your wired network. Then Windows will act as a mini-DHCP server (or will pass through some information so that the Beaglebone can reach DHCP on the larger network). In the file /vim/etc/interfaces, uncomment the two lines auto eth0
and iface eth0 inet dhcp
. Save the file, and run the commands ifdown eth0
and ifup eth0
until ifup succeeds in obtaining an IP address. (Sometimes the first attempt, sometimes much more than this.)
Prelab
There was no opportunity for prelabbing this week
Introduction
In this lab, we are going to build a simple camera which will take pictures when commanded via a pushbutton. The pictures can then be viewed on the LCD display of the Beaglebone.
Part 1: Getting started.
To begin with, we will need to make a few modifications to the setup on our Beaglebone, as some of the packages we need are not installed in the system.
To begin, log into your Beaglebone and connet it to the internet (e.g. ping google.com
should work). Issue the following commands in sequence to install appropriate libraries:
sudo apt-get update sudo apt-get install gpicview sudo apt-get install libopencv-dev
The update command will update the package listing on your Beaglebone to reflect the most current packages which are available. The install commands will install the gpicview image viewer program and the OpenCV development libraries, which are necessary to access the camera.
Once you have completed this task, unplug the power to your Beaglebone and plug in the LCD display and the USB camera in your kit. (The camera doesn't automatically show up unless you reboot the beaglebone. Feel free to try. I did.) Reboot your Beaglebone linux machine and open up a terminal connection. Issue the command lsusb. This command will list the usb devices attached, and should result in the display shown below:
Bus 001 Device 002: ID 046d:082d Logitech, Inc. Bus 001 Device 001: ID 1d6b:0002 Linux Foundation 2.0 root hub Bus 002 Device 001: ID 1d6b:0002 Linux Foundation 2.0 root hub
Compiling our first code
The first code that you will use is a preconstructed program. This program will use openCV to capture an image from the camera and to filter it into greyscale and using an edge detection algorithm. Ultimately, we will only be using the image capture portion of this in our final project, but we want to see each of them working.
Download the file boneCV.cpp. This file will use a library called openCV to capture an image and write it into a file. Transfer this cpp file to the Beaglebone and issue the command
g++ -O2 `pkg-config --cflags --libs opencv` boneCV.cpp -o boneCV
If you type this command, be sure to type a letter O instead of a digit 0 for -O2. Also, use the accute accent quotes `...` instead of single quotes '...'
.This will compile the program on the Beaglebone. After compilation is completed, execute the program. You will see that three images are created. Open them in gpicview using the touchscreen. (When plugged in, the touchscreen automatically opens to /home/debian/Desktop, without needing to enter any login info. Once there, you can easily navitage to any subfolder of the Desktop. Double-click on an image to open it with gpicview.) Examples are shown in the Figure below
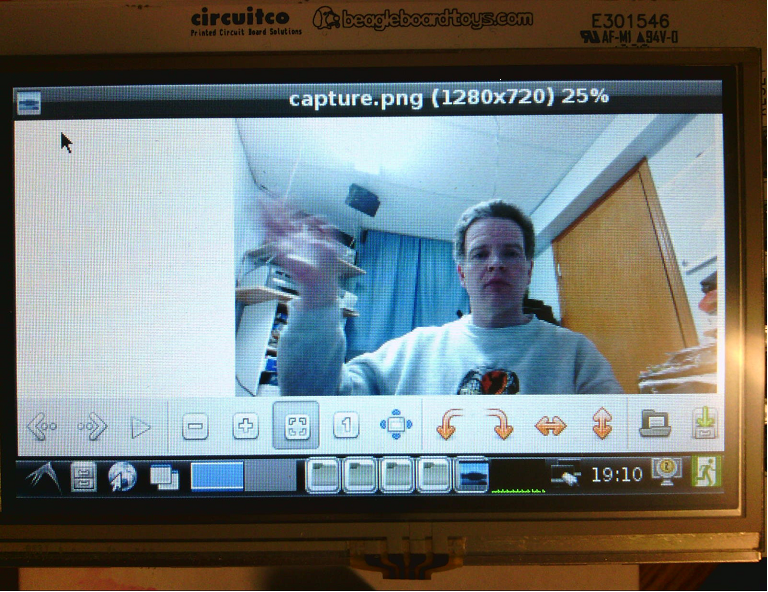
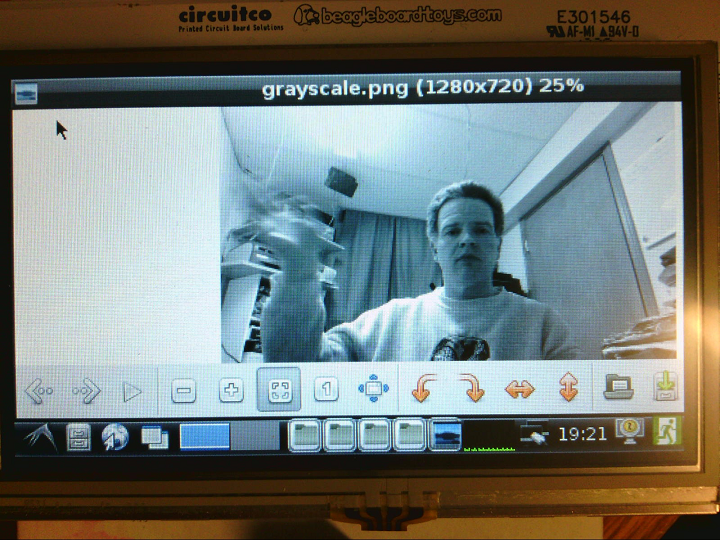
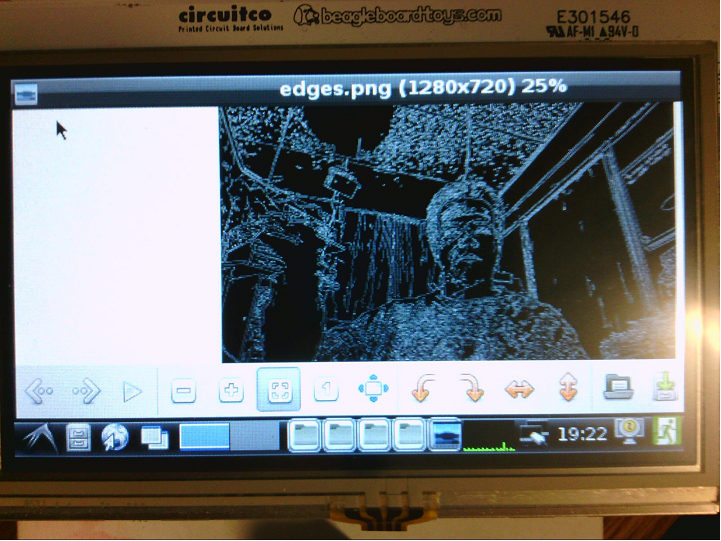
Taking a snapshot of your virtual machine
Before going further, we want to take a snapshot of your virtual machine. That way, if there are problems, we can easily recover the machine back to the previous state. To do this, select the snapshots tab in the upper right and click on the "take snapshot" icon that appears to the left. Provide a meaningful name for the snapshot and a description prior to pressing OK. This will take a few seconds, but now it is possible to revert back to the previous state if something goes wrong.
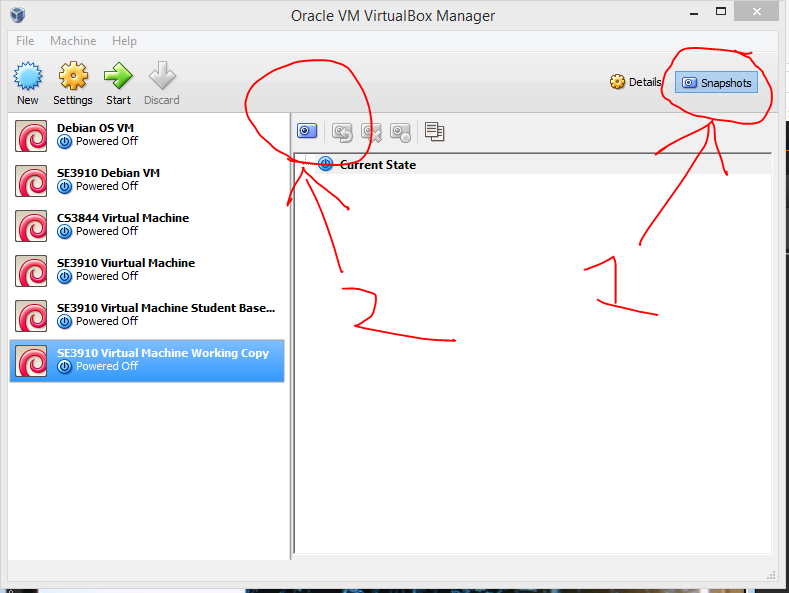
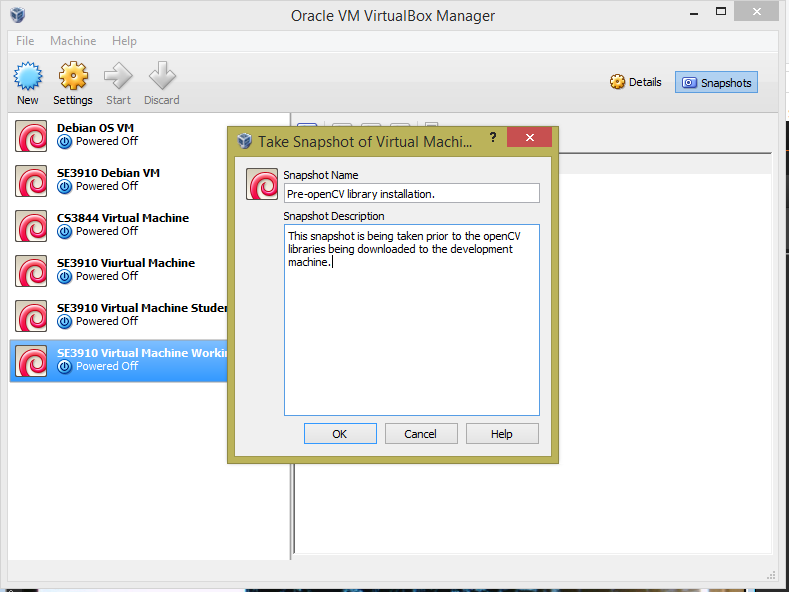
Installing header files for the code
Due to some technical issues with the Beaglebone development environment and the currently available cross compilation libraries, we are not able to link our code in the cross compiled environment. We can, however, use Eclipse to write and compile our code locally, getting a great deal of the benefit of the IDE's error-checking abilities. We will, however, be forced to re-compile and link the code on the Beaglebone to create the working executable.
To start, download the tar file lab5update.tar.gz. This file contains a script and an install set. Extract the tar file somewhere reachable by the VM and, from the VM, run the script lab5setup.sh as root. This will install the library header files in the appropriate locations for the tools to work properly. To run lab5setup.sh, use
sudo ./lab5setup.sh
(You can look into this plain-text file to see what the script does, if you are curious.)
Developing your code
Once the libraries are installed, you will be developing a camera application. This application will use openCV to capture images and store them to files. You will be able to vary the size of the image captured. Additionally, you will be able to set the starting number for images that are captured. For this exercise, you will be capturing png files, though other files can be captured as well.
The program has these 3 basic requirements
- Requirement R1: When the user presses a pushbutton, the camera shall take their picture and store it into a png file.
- Requirement R3: The program shall turn on an LED while the camera is taking the picture.
- Requirement R4: The program shall turn on a second LED while the actual file write of the image occurs.
- Requirement R2: The input and output GPIO port numbers (one input switch and the two outputs for R3 and R4 above), and the height and width of images captures shall be variable based on a parameter passed in on the command line.
- Requirement R5: The program shall automatically increment the number of the image file saved as each picture is taken.
- Requriement R6: When run without parameters, the program shall print out the arguments in the order it expects them.
Your program can take an arbitrarily large number of pictures. It is OK if the user needs to use Ctrl-C to exit.
Below is a template UML diagram. Your code does not need to match this exactly, but this is a good starting point for your system design. You will want to perform your setup for the camera once in the constructor, and then simply capture frames and write them out in the takePicture function.
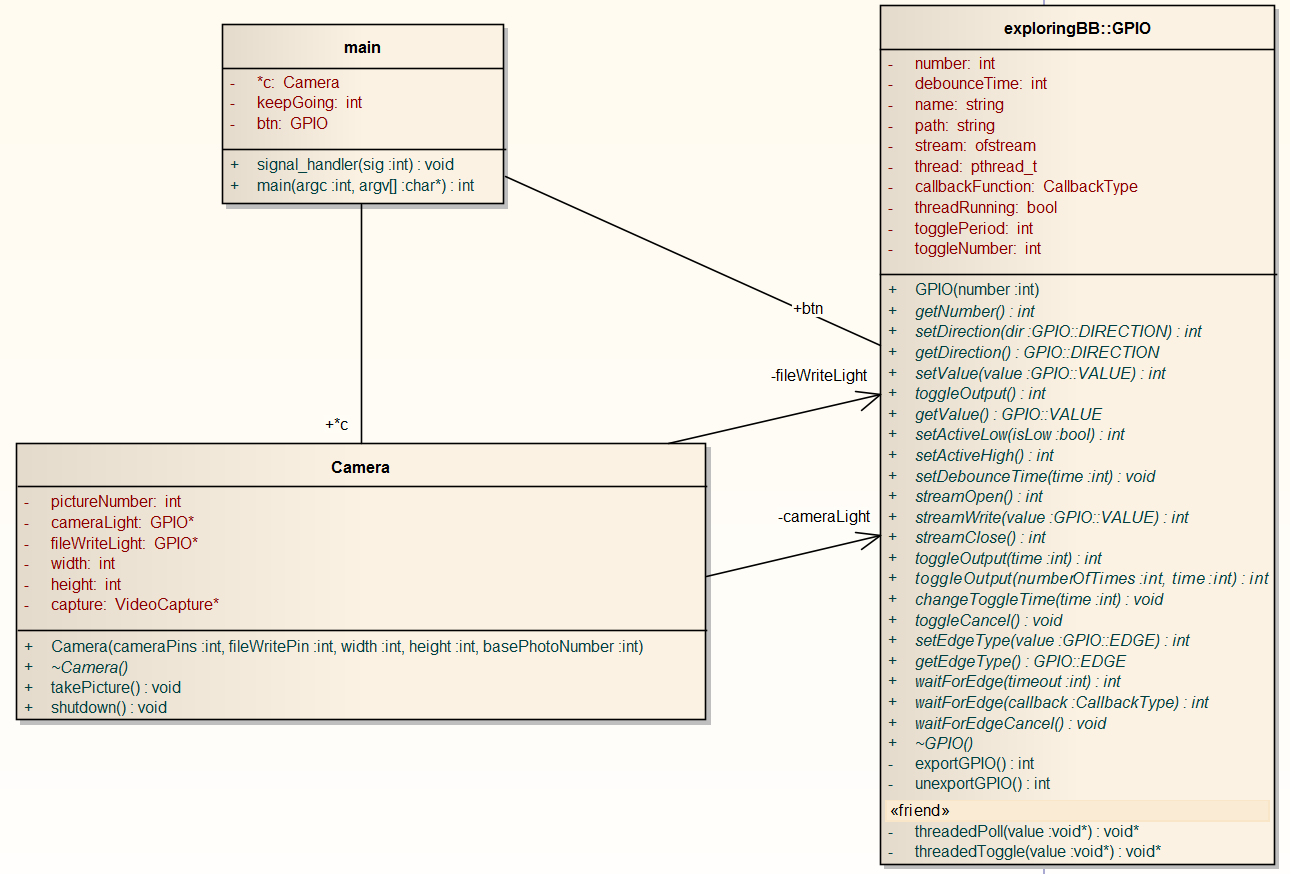
Compiling and Linking your code
You will be able to compile your code locally. However, as was stated previously, libraries make it difficult to link your code in a cross compiled environment. To get around this, create a directory on your Beaglebone for your code to reside (e.g. /home/debian/Desktop/camera
) and transfer it via ftp to your Beaglebone.
To do this, open up a shell on your Linux Virtual Machine (VM) and issue the command
sftp debian@beaglebone.local
to connect to the Beaglebone. Once connected, issue the command cd camera
to change into the remote camera directory and then put *
to transfer all of the files from the local machine to the remote machine.
(Alternatively, you can use a command like scp *.cpp *.hpp debian@192.168.7.2:Desktop/camera/
if you have Cygwin installed)
Now open up an ssh connection to the Beaglebone. Change into the directory containing your code and issue the command
g++ -O2 `pkg-config --cflags --libs opencv` *.cpp -o camera
to compile and link the program on the Beaglebone.
(If you have .cpp files that you don't wish to compile, you will have to name all the cpp files that you DO want to compile instead of just using *.cpp.)
Measuring how long it takes to snap a photo and how big they are
As a last piece, we want to know how long it takes for the photo to be snapped. Connect your two oscilloscope channels to the two LED pins and measure how much time it takes for a picture to be taken, and how long it takes for the picture to be written to disk. Measure the times for various sizes and plot the relationship between size (i.e. number of pixels) and the time it takes to take the picture, the time it takes to store the picture, and the size of the picture in bytes. Make sure to vary your picture sizes from 320 * 240 to 1920 * 1080.
After you are done with this, trying changing the picture format to jpg and repeat the experiment. Is there a difference?
Deliverables / Submission
Use the SE3910Lab5Report.docx template when writing your report.
If you have any questions, consult your instructor.
Acknowledgement: This lab originally developed by Dr. Schilling.
Due: Week 6, Monday, 11pm
Submission Instructions for Dr. Yoder
Submission due 11pm, Monday of Week 6
Please upload a screenshot containing two traces — one for the button input and one for the LED output from the beaglebone, showing the latency between when the button is pressed and the LED changes its state.
Also include a text file including the latency as you read it from the screenshot. Put your name and the date at the top of this text file.