You will need headphones or speakers for this lab.
This assignment is a team assignment. Please work in teams of two unless approved by the instructor. I'm likley to approve working individually on this lab as well.
Introduction
In this lab, you will create a GUI using the Qt library and IDE. The application will be a talking clock with customizable photo display.
Prelab
- (Required before lab)
- Install Qt Creator in your VM
- (Recommended before lab)
- Write your Qt application
- Install the Qt Creator within the chroot on your BBB (BeagleBone Black)
- Compile your application within the chroot on the VM
- Install the Qt Creator libraries on the BBB, making sure there is enough space and resizing the BBB's drive before you run the commands if needed.
Installing Qt Creator, etc. on VM (can be done with just VM on network)
This lab involves a few significant changes to your virtual machine environment. Ideally, you should do this on a wired connection, as there are significant downloads to be made. Be sure the "cable connected" box is checked under Network Settings (as usual). You should be able to ping Google before running these commands.
Before installing qtcreator, we need to install some packages on which it depends. This is to work around a bug in these packages in the current version of debian.
Edit the file /etc/apt/sources.list, e.g.:
sudo nano /etc/apt/sources.list
To /etc/apt/sources.list, add the line
deb http://ftp.debian.org/debian jessie-backports main
at the end of the file.
Run the command:
sudo apt-get update
Now install these libraries as recommended on this stack overflow post:
sudo apt-get -t jessie-backports install libegl1-mesa libegl1-mesa-drivers libgl1-mesa-dri libgl1-mesa-glx libglapi-mesa libgles1-mesa libgles2-mesa libglu1-mesa libopenvg1-mesa libtxc-dxtn-s2tc0 libwayland-egl1-mesa mesa-utils mesa-utils-extra libva-drm1
At this point, you can proceed with the instructions below for installing Qt onto your Virtual Machine (outside the change root)
On your Virtual Machine, issue the following commands in order.
sudo apt-get update sudo apt-get install qtcreator sudo apt-get install qt4-dev-tools
... install qtcreator
will install the QT creator application and libraries on your machine.
qt4-dev-tools
install to install the qmake tools which are needed to manually build qt applications on your virtual machine.
Next, start the change-root, and within the changeroot, run these same commands.
You will need about 200MB of extra space on your Beaglebone to install the libraries needed for this lab. If your Beaglebone is Revision C, all is well. To check how much extra space is available on your beaglebone, use the df -h
command.
If, as in the warning above, your df -h command does not show enough space on your BeagleBone's drive, follow these instructions to create enough space:
- Ensure the BeagleBone can connect to the internet (
ping google
) - Run these commands to repartion the BeagleBone into all the available space.
- cd /opt/scripts/tools
- git pull
- ./grow_partition.sh
- sudo reboot
Finally, connect to your BBB and run these same commands. (Your BBB will need to be connected to the internet.)
Before booting the BBB with the LCD attached, you will need to edit /boot/uEnv.txt as described in Lab5. You can do this while working the prelab.
Building your basic applications
Once you have the QT Creator installed, you are to build your first application, a simple clock. Launch QT Creator through Applications Menu->Development->QT Creator. In your vm shared folder (e.g. /media/sf_vmshare/), create a qt folder, and within it a Lab6DigitalClock folder (e.g. /media/sf_vmshare/qt/Lab6DigitalClock). In the Lab6DigitalClock folder, create each example file from the Digital Clock Example and copy the contents from the webpage into the file. (You can do this from the Windows side through the shared folder. Be sure to give each file exactly the same name as in the example.) Launch QtCreator from Applications Menu -> Development -> Qt Creator. From QtCreator on the VM, open the .pro file with the File->Open File or Project menu/dialog. Accept the default import settings. I had to do this twice Spring 2016 to get it to work. If asked, click through to set up the Qt build environment. Run the project by clicking on the green arrows. You should see the clock running within your VM something like this:
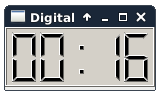
Once you have completed this, compile and run the Simple Image Viewer example in the same way. This will walk you through constructing a simple QT image viewer. Again, verify that the code runs on your virtual box machine and then try running it on your Beaglebone.
The Main event (Writing your source code)
Once you have gone through these two tutorials, combine the widgets which are created in the first and second tutorials together with a pushbutton to create the application shown below. If the user clicks on the speak the time button, the application should make a system call to speak a message like "The current time is now 12:15:45."
While developing the application in Qt, print to standard out instead of running any commands that don't work on the VM. For example, while testing your prelab, simply print the message like "The current time is now 12:15:45" (with the current time) to the console rather than making a system call.
You do NOT need to capture images from the camera in this lab, but you DO need to show an image in your app.
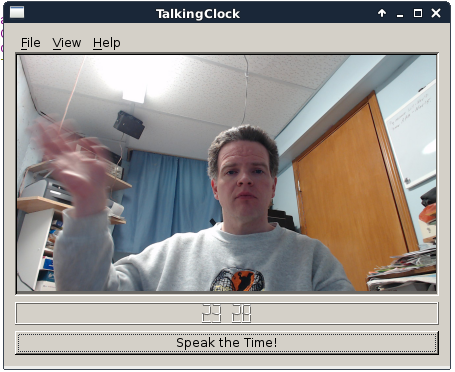
In-lab testing
Setting up a VNC server with virtual screen (tightvncserver)
Spring 2017: You do NOT need to get your BBB to talk with text-to-speach. Instead, light up an LED or simply print to standard out when the button is pressed.
Instead of using the LCD, you can run a VNC server on your BeagleBone.
- On the BeagleBone, run the command tightvncserver. This is already installed by default. When asked, come up with a reasonably-secure password that you will use when connecting to your BeagleBone (especially if you have changed the root password...)
- On the Virtual Machine,
- sudo apt-get install ssvnc
- ssnvcviewer 192.168.7.2:1
When you run the viewer on the Virtual Machine, you should see a window with a desktop showing up. This is the desktop of your BeagleBone. Any files placed in /home/debian should show up on this desktop. Place your application here and double-click on it to run it.
Setting up a VNC server to share the LCD screen with a client on your VM
If you want to have the video show up on the LCD, but also want to use VNC to log into the X11 window remotely, you need a different VNC server on the Beageblone.
On the beagelbone,
- Install the x11nvc server with
apt-get install x11vnc
- Change to user debian with
su debian
- Change into the debian user's home directory with
cd
- Run the x11vnc server with
x11vn
- Look at the output — does it warn that it cannot connect to :0? If not, it may have been successful!
On the virtual machine
- sudo apt-get install ssvnc #If you haven't already done this.
- ssnvcviewer 192.168.7.2:0
Note that this time we are connecting to display 0, the default display.
I have not yet been able to confirm that this will allow you to get past the login screen. If you try it, please report your results.
If you are getting the login sceeen then you have to run some more commands to get x11vnc to work on the BBB
- su debian
- cd # To Debian's home.
- sudo chmod -R o+rwX /var/run/lightdm
- x11vnc -auth /var/run/lightdm/root/:0
If your ssh connection is flakey
you may want to run the final command with nohup so it keeps running even if your ssh is disconnected.
- If you get past login screen without VNC:`
- nohup x11vnc&
- tail -n +1 nohup.out
- If you need VNC to get past login screen:
- nohup x11vnc -auth /var/run/lightdm/root/:0 &
- tail -n +1 nohup.out
If you want to recalibrate the screen move /etc/pointercal.xinput
to /etc/pointercal.xinput.hidden
(so the computer won't find it, but you can copy it back later if something goes wrong...) and reboot the BeagleBone.
Appendix: A variation on getting the sound to work
The final command may need to be changes based on how your soundcard ends up being setup. Dr. Schilling used mplayer -ao alsa:device=hw=5 test.wav
. The command aplay -l
(with a lower-case L) may help to find the right hardware number. (More details on superuser) For example, when I ran it it produced something like ...
**** List of PLAYBACK Hardware Devices **** card 1:
I believe the card number corresponds to the nuber you put after hw= in the mplayer command. This stack overflow question may have more info.
Deliverables / Submission
Submit a printout of your source files and (if not demoed in person) a picture/screenshot of your image-clockp GUI running on the BeagleBone.
Please print this checklist and staple it on top of your source files.
If you have any questions, consult your instructor.
Hints
Here are a few websites you might want to look at if you are stuck:
- http://ldc.usb.ve/docs/qt/tutorial-t5.html
- https://wiki.qt.io/How_to_Use_QPushButton
- http://exploringbeaglebone.com/chapter12/
- https://www.youtube.com/watch?v=PQEBoftbtVQ
Acknowledgement: This lab originally developed by Dr. Schilling.